The Need
I've been looking for a DEX with an orderbook where I could list my RWA token for a fixed price fundraising, but couldn't find one. Here's my use case:
- Initial Sale: I want to tokenize a real estate development project and sell tokens at a fixed price to raise construction funds
- During Construction (1+ years): Token holders should be able to trade their tokens at prices they choose
- Project Exit: After selling the property, I want to place a single large buy order at a fair price so all token holders can exit at the same rate
- Capital Efficiency: All raised funds should be available for business needs (land purchase, materials, labor) without locking any portion in liquidity pools
While AMM-based DEXes like Uniswap have become the standard for DeFi, they aren't suitable for this type of RWA token because:
- AMM pools would cause price fluctuations, making it unfair for initial buyers and final exit
- They require locking significant capital in liquidity pools
- Price discovery mechanism doesn't match RWA trading patterns RWA trading often requires more precise price control and doesn't need the high-speed execution that AMMs provide. This post explores a simple approach to building an on-chain orderbook DEX specifically designed for RWA tokens.
The Problem
Traditional orderbook DEXes are often considered impractical on-chain due to:
- High gas costs for order matching
- Complex order matching logic
- Front-running concerns
- Speed limitations of on-chain execution
However, for RWA tokens, these limitations are less critical because:
- Trading velocity is lower
- Price precision is more important than execution speed
- Front-running is less profitable due to lower volatility
- Gas costs are more acceptable given higher transaction values
The Solution: Simple On-Chain Orderbook
We can build a simplified on-chain orderbook DEX with these key features:
Core Design
- One smart contract per token pair
- Fixed price steps to simplify matching
- Price-indexed mapping for orders
- Off-chain order matching calculation
- On-chain order execution
- Factory contract for easy deployment of new pairs
Data Structure
// Price -> [Orders at this price level]
mapping(uint256 => Order[]) public buyOrders;
mapping(uint256 => Order[]) public sellOrders;
struct Order {
address maker;
uint256 amount;
bool isBuyOrder;
}
Key Functions
- Create Order
- Takes price and amountValidates price stepTransfers tokens to contractStores order in mapping
- Take Order
- Takes existing order's price and indexTransfers tokens between partiesRemoves fulfilled order
- Cancel Order
- Only original maker can cancelReturns locked tokensRemoves order from mapping
Price Steps
- Each pair has a fixed minimum price step (e.g., 0.01)
- Prices must be multiples of this step
- Larger steps mean fewer possible price levels
- Reduces storage costs and simplifies matching
Frontend Integration
- JavaScript frontend calculates best matching orders
- Can easily scan available orders at each price level
- Simplified order matching due to price step structure
- Clear view of liquidity at each price level
Advantages
- Simple and predictable gas costs
- Easy to understand and audit
- Precise price control for traders
- No impermanent loss
- Perfect for RWA tokens where speed isn't critical
- Ideal for fixed-price token sales and fair exits
- No capital locked in liquidity pools
- Supports both fixed-price primary market and variable-price secondary market
- Fair and equal treatment of token holders during exit events
Gas Optimization
- Fixed price steps reduce storage complexity
- Direct price indexing for quick lookups
- Batch operations possible for efficiency
- Simple matching logic reduces computation
Limitations
- Higher gas costs than AMMs
- Not suitable for high-frequency trading
- Less capital efficient than AMMs
- Requires more active market making
Future Improvements
- Partial order filling
- Better order prioritization
- Multiple token pair support in single contract
- Gas optimization for bulk operations
- Integration with liquidity provider incentives
Conclusion
For RWA tokens, a simple on-chain orderbook can provide better price discovery and more precise trading than AMM-based solutions. While this approach wouldn't work well for high-frequency trading tokens, it's perfectly suited for the RWA market where trade frequency is lower and price precision is more important than execution speed.
The implementation is straightforward enough that it can be built, audited, and maintained by small teams, making it an attractive option for RWA token projects that need a custom trading solution.
What do you think about this approach? Would love to hear your thoughts and suggestions for improvements.
[link] [comments]
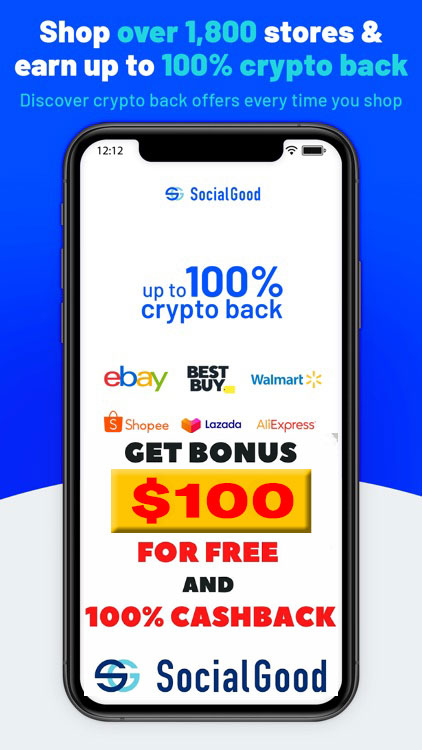
You can get bonuses upto $100 FREE BONUS when you:
💰 Install these recommended apps:
💲 SocialGood - 100% Crypto Back on Everyday Shopping
💲 xPortal - The DeFi For The Next Billion
💲 CryptoTab Browser - Lightweight, fast, and ready to mine!
💰 Register on these recommended exchanges:
🟡 Binance🟡 Bitfinex🟡 Bitmart🟡 Bittrex🟡 Bitget
🟡 CoinEx🟡 Crypto.com🟡 Gate.io🟡 Huobi🟡 Kucoin.
Comments